The singleton pattern ensures class has only one instance, and provides a global point of access to it. 1
You can see an example code from this video.
singleton pattern
It’s one of design pattern described by GOF (Gang of Four).
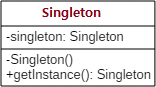
There are many objects we only need one.
In example, There are such as device driver, data repository and logging.
I always use Singleton rather than a global variable because the code in my project is much more concise.
This chapter explains how to implement singleton pattern with python with example code.
And I designed it to be thread safe because I sometimes use multi treading in python.
Singleton.py
I made Sington
type which is able to create and hold an instance.
Please refer this page if you want to understand metaclass
and type
.
from threading import Lock
class Singleton(type):
_instance = None
_lock = Lock()
def __call__(cls, *args, **kwargs):
with cls._lock:
if not cls._instance:
cls._instance = super().__call__(*args, **kwargs)
return cls._instance
SingletonClass.py
This is an sample Singleton class to hold variables and to provide interfaces.
This SingletonClass
has a name
variable that is set when class is instantiated.
from Singleton import Singleton
from threading import Thread
class SingletonClass(metaclass=Singleton):
def __init__(self, name):
self.name = name
def run(value):
singleton = SingletonClass(value)
print(singleton.name)
if __name__ == '__main__':
processa = Thread(target=run, args=('ACLASS',))
processb = Thread(target=run, args=('BCLASS',))
processa.start()
processb.start()
Test Result
I created 2 threads calling of each SingletonClass(value)
.
Even if you call SingletonClass
with 'BCLASS'
as the name
, both objects show the name
of the object created at first.
ACLASS
ACLASS
What is the Start Python
series
Most beginners start programming by printing ‘Hello world!’ and learning the syntax, but we know that a project requires more things.
So, I would like to introduce a code snippet what I met in my actual project.
I will not use framework such as Django because people have difference environment and use cases.
Please let me know if you need further information about this article.
- Freeman Robson, Head First Design Patterns, O’REILLY, 2014, p179 [↩]